UICollectionViewをコードのみでの実装
- エラー処理していません
- ほぼ最小構成ですが削れるところがあるかもしれません
環境
- Xcode 11.4
- Swift 5
完成形
正方形のセルを横5列に並べました
色はわかりやすいようにつけただけで意味はありません
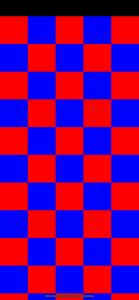
解説
解説いらない方は最下部にコード全文載せてます
まずはViewControllerに「UICollectionViewDataSource」を継承とUICollectionViewの変数を作成
class ViewController: UIViewController , UICollectionViewDataSource {
var collectionView: UICollectionView!
/* 省略 */
ViewDidLoadの中でレイアウトの作成と「CollectionView」をaddsubview
CollectionViewはviewのサイズで作成しました
override func viewDidLoad() {
super.viewDidLoad()
let flowLayout = UICollectionViewFlowLayout()
flowLayout.itemSize = CGSize(
width: self.view.frame.width / 5,
height: self.view.frame.width / 5
)
flowLayout.minimumInteritemSpacing = 0
flowLayout.minimumLineSpacing = 0
flowLayout.sectionInset = UIEdgeInsets(top: 0, left: 0, bottom: 0, right: 0)
collectionView = UICollectionView(
frame: self.view.frame ,
collectionViewLayout:flowLayout
)
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: "Cell")
collectionView.dataSource = self
self.view.addSubview(collectionView)
}
「UICollectionViewDataSource」で必要なものを最低限追加します
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
//セルの数
return 100
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell", for: indexPath)
//奇数偶数で背景の色変更
if(indexPath.row % 2 == 0){
cell.backgroundColor = .red
}else{
cell.backgroundColor = .blue
}
}
コード全文
import UIKit
class ViewController: UIViewController , UICollectionViewDataSource {
var collectionView: UICollectionView!
override func viewDidLoad() {
super.viewDidLoad()
let flowLayout = UICollectionViewFlowLayout()
flowLayout.itemSize = CGSize(
width: self.view.frame.width / 5,
height: self.view.frame.width / 5
)
flowLayout.minimumInteritemSpacing = 0
flowLayout.minimumLineSpacing = 0
flowLayout.sectionInset = UIEdgeInsets(top: 0, left: 0, bottom: 0, right: 0)
collectionView = UICollectionView(
frame: self.view.frame ,
collectionViewLayout:flowLayout
)
collectionView.register(UICollectionViewCell.self, forCellWithReuseIdentifier: "Cell")
collectionView.dataSource = self
self.view.addSubview(collectionView)
}
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 100
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell", for: indexPath)
if(indexPath.row % 2 == 0){
cell.backgroundColor = .red
}else{
cell.backgroundColor = .blue
}
return cell
}
}