よく使われるUITableViewの基本的な利用方法
UITableViewの基本的な使い方
Stroyboardを使わずにコードのみ、オートレイアウトもコードで行っています
class ViewController: UIViewController {
var tableView:UITableView!
let items:[String] = [
"Cell 1",
"Cell 2",
"Cell 3",
"Cell 4",
"Cell 5",
]
override func viewDidLoad() {
super.viewDidLoad()
self.tableView = UITableView()
self.tableView.delegate = self
self.tableView.dataSource = self
self.tableView.translatesAutoresizingMaskIntoConstraints = false
self.view.addSubview(tableView)
self.tableView.topAnchor.constraint(equalTo: self.view.topAnchor).isActive = true
self.tableView.leadingAnchor.constraint(equalTo: self.view.leadingAnchor).isActive = true
self.tableView.trailingAnchor.constraint(equalTo: self.view.trailingAnchor).isActive = true
self.tableView.bottomAnchor.constraint(equalTo: self.view.bottomAnchor).isActive = true
}
}
extension ViewController : UITableViewDelegate , UITableViewDataSource {
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = UITableViewCell(style: .value1, reuseIdentifier: "cell")
cell.textLabel?.text = self.items[indexPath.row]
cell.detailTextLabel?.text = indexPath.row.description
return cell
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return self.items.count
}
}
これを実行すると下記のような画面が表示されます
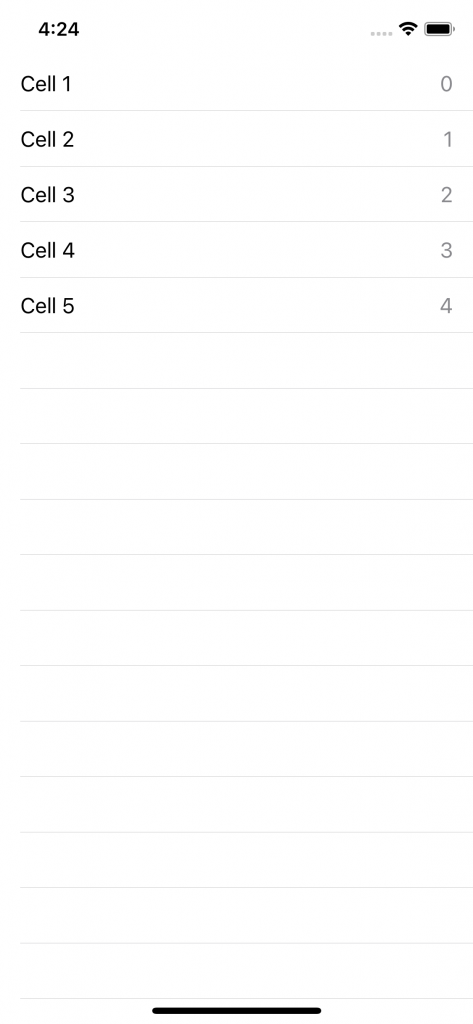
TableViewのセルをタップして検出する
セルのタップを検出するにはDelegateに下記を追加します
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
print("セル\(indexPath)をタップ")
}
タップされると上記が実行されます
UITableViewの基本的な使い方は以上です